In this post and video, I am comparing 2 mostly used techniques of Client and Server interaction – Sessions Vs JWT For Authorization. The two approaches differ in where the authorization information is stored. In the session based approach, the information is stored on server-side, whether in memory or in database etc. In the case of JWT, a token is generated upon successful authentication and sent to the client. The client must send the token in request header for any protected end points. We will also create a working demo of JWT based interaction using Express Server and jsonwebtoken.
Session Based Client Server Interaction
As shown below, the session based Client and Server interaction starts with a client sending Authentication request. Upon successful authentication, Server generates a session for client, stores on server side (in memory Or database) and sends session information in a cookie. The client will send cookie with every subsequent request. The server will validate the information sent in the cookie against what is stored on server side. If successfully validated, the access is granted to the protected end point.
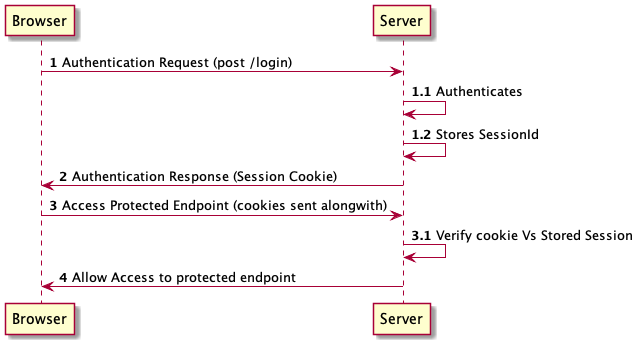
Common Issues With Sessions
In the session based client server interaction, most of the issues arise with the information stored on Server side. In case of Single server, the server going down would lose all the client’s sessions, In case of multiple servers storing sessions locally, you need to have a load balancer with Sticky Session, and in case of a external caching like Redis, we introduce a single point of failure. Let’s see how JWT addresses these issues.
What is JWT?
JWT is short for JSON Web Token. It is is an open standard that defines a compact and self contained way for securely transmitting information between parties. It’s trusted as the issuer Digitally Signs it. It can be signed using a secret Or Public/Private key pair. One thing to note that JWT is not for Authentication.
JWT is useful for Authorization And Information Exchange.
Authorization is common use for JWT token. After a user is Authenticated, each subsequent protected route / service request can include JWT in Authorization Header.
Information Exchange, can be used as the token can be created using Public/Private key pair, hence the Sender and Receiver trust each other
JWT Based Client Server Interaction
As shown in the below, the JWT based Client and Server interaction starts with a client sending Authentication request. Upon successful authentication, Server generates a JWT token for client and sends the token in response either header on body. The client will send the token in Authorization header with every subsequent request. The server will validate the token. If successfully validated, the access is granted to the protected end point.
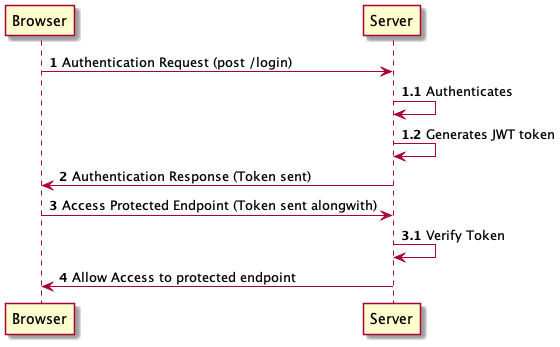
JWT Structure
Every JWT token has 3 parts: Header, Payload and Signature. Each one is Base64 encoded and concatenated with a period (.).
Sample Token
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpZCI6MTAwMSwibmFtZSI6IkdpcmlzaCBKYWp1Iiwid2Vic2l0ZSI6Im15Q2xvdWRUdXRvcmlhbHMuY29tIiwiaWF0IjoxNjI0NDg2MzUzfQ1.6CQATmjLPlDx41mSGXAJ9CHZu0XRV6T0J6b79r8BYvg
You can copy the JWT token on jwt.io and see the following decoded information.
Decoded Token Sample
Header
{
"alg": "HS256",
"typ": "JWT"
}
Payload
{
"id": 1001,
"name": "Girish Jaju",
"website": ”MyCloudTutorials.com",
"iat": 1624486353
}
Signature
HMACSHA256( base64UrlEncode(header) + "." + base64UrlEncode(payload), ) secret base64 encoded
Does JWT solve everything?
JWT is definitely an improvement on the Session based approach. Since it’s not stored on the server, any server can serve the client’s request, making the solution much more scalable, as it’s not dependent on routing the request to a particular server.
But Does JWT solve everything? Well, of course not.
Some of the common issues that we may come across while using JWT are:
- Stolen JWT
If a JWT ends up in the wrong hands, a malicious user can access a protected end point by sending the JWT in the Header. As long as the JWT token validation is successful, Server will honor the request.
How do you prevent such a thing?
We can maintain a BLACK LISTED JWT list. This will be a maintenance nightmare.
We can also change the SECRET KEY on the server, which will make all the olders JWT tokens invalid. Although not an ideal solution, but it would prevent the damage that a hacker can inflict upon your system if he/she gets hold of a valid JWT token.
- JWT with Longer Expiry:
Longer the expiry of JWT token, more are the chances that it may get into wrong hands. You should design your token’s expiration carefully and refresh the tokens.
Code for this demo is available at https://github.com/mycloudtutorials/jwt-demo
Demo – Express Server and Node JS
Please check the following Youtube video, where I am discussing the issue in detail, Plus the demo of creating the Express server and protecting the endpoints using JWT token, step by step.